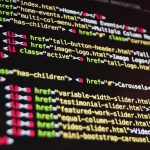
Powershell Code Script for Mass BlogTalkRadio Show Downloads.
Recently, I found that the sheer cost of BlogTalkRadio’s hosting plan was something I was no longer prepared to foot the bill for. I realized I would need to host my shows elsewhere, and decided to download them all. But, with over 300 shows in the lineup, I had a big problem on my hands to get them all download. So… it was time to write a script!
It turned out that there was a toolbox for reading RSS feeds that was perfect for the job called “PSWebToolbox.” This library contains everything you will need and is the lynch-pin for this custom script of mine.
I only needed to use this code the single time to do the job needed of it, but felt it was a crying shame to let it sit idle when so many other BlogTalkRadio show hosts likely would lose all their shows unless they had a script like this to use to help download all of their shows. So… I am giving it to them in the spirit of friendship.
Here is my custom Powershell script. I am releasing it as a script under CC BY-NC 4.0 non-commercial license for individuals and groups to use to copy their files. NO COMMERCIAL USE. Full attribution to the author is required.
The only three places to make changes for your needs are highlighted in bold. The directory for the destination files and the URL of the site feed. Note that this can be used in other types of downloads for site feeds, but WILL require modifications for non-BlogTalkRadio sites, since the date/show/folder structures likely differ. The “999” is a count of the shows. You can leave it as-is, or change it if you know the exact number of shows. I’d recommend you get the show count so the script runs through cleanly with the exact count. You can also comment out the MP3 download line to have the script create ONLY the show folder structures if you need that to happen. See line below:
#Invoke-WebRequest -Uri $fullBTRmp3FilePath -OutFile $finalFullFile
You are expected to realize that this script is release “as-is” and I make ZERO guarantees that it will work for your needs. Like many things in life, what worked before may not work now, and you may need to tweak it accordingly to meet the need.
Final recommendation:
I do heartily recommend you visit your show link in a browser and add “.rss” to the end of the URL. This will show you your entire show feed with episodes and all the metadata about them. I would scrape a copy of this and save it for perpetuity, since this is THE complete history of your shows and episode titles/descriptions. You won’t get it back once you end your subscription with BlogTalkRadio.
Make the needed changes to use it on your Windows system and turn it loose to download your shows! Enjoy!
Script Code
# BlogTalkRadio MP3 downloader for all shows
# Writes full folder paths based off the URL pathname.
# Author: Jon Almada
# This script will create a folder structure and copy the MP3 file from BlogTalkRadio to match the exact
# structure of the URL. This script works by reading the RSS file from BlogTalkRadio for a given show URL
# for the RSS feed and then creating the necessary folder structures to match the
# Required Powershell Module
# You will need to install the following module with command: “Install-Module PSWebToolbox”
# to allow the Get-RSSFeed to work. Note that you need to change the destPath, feed URL and the “-Count”
# as required to download all of the files.
# https://github.com/EvotecIT/PSWebToolbox to learn more about PSWebToolBox.
$destPath = “D:/Example/mysitefeedlink”
# Use -All for all files
$Blogs = Get-RSSFeed -Url ‘https://my.blogtalkradio.com/mysitefeedlink.rss‘ -Count 999
if (-not $StatusBlogs) {
$StatusBlogs = [ordered] @{}
}
foreach ($Blog in $Blogs) {
if ($StatusBlogs[$Blog.Link] -eq $true) {
continue
}
#Construct the full downloadable MP3 file path from BTR
$fullBTRmp3FilePath = $Blog.Link + “.mp3”
#Strip the https://blogtalkradio.com off the path
$URLFullPath = $Blog.Link.Substring(34)
# Get the MP3 file name
$fileName = $URLFullPath.Substring($URLFullPath.LastIndexOf(‘/’)+1)
#remove the file name from the path
$filePath = $URLFullPath.Trim($fileName)
#Strip the first / from the filePath
$filePath = $filePath.Substring($filePath.IndexOf(‘/’)+1)
#Create the needed MP3 file
$mp3File = $fileName + “.mp3”
$finalFullFile = $destPath + “/” + $filePath + $mp3File
#Write-Output $destPath/$filePath
Write-Output $finalFullFile
#create the directory
New-Item -Path $destPath/$filePath -ItemType Directory
#download the MP3 for this file
Invoke-WebRequest -Uri $fullBTRmp3FilePath -OutFile $finalFullFile
}